Portfolios allow for efficient pricing and risk. The same principles in the in the basic risk and pricing tutorials can be applied to portfolios. Pricing and risks can be viewed for an individual instrument or at the aggregate portfolio level
info
Note
Examples require an initialized GsSession and relevant entitlements. Please refer to Sessions for details.
from gs_quant.session import Environment, GsSession
from gs_quant.common import PayReceive
from gs_quant.instrument import IRSwaption
import gs_quant.risk as risk
import datetime as dt
swaption1 = IRSwaption(PayReceive.Receive, '5y', 'EUR',
expiration_date='3m', name='EUR3m5y')
swaption2 = IRSwaption(PayReceive.Receive, '5y', 'EUR',
expiration_date='6m', name='EUR6m5y')
# Create Portfolio w/ swaptions
from gs_quant.markets.portfolio import Portfolio
portfolio = Portfolio((swaption1, swaption2))
portfolio.resolve()
# Calculate Risk for Portfolio
port_risk = portfolio.calc(
(risk.DollarPrice, risk.IRDeltaParallel, risk.IRVega))
# View Instrument Level risk for portfolio
port_risk[risk.IRDeltaParallel]['EUR3m5y']
Output:
date 2020-02-13 -293.405359 dtype: float64
# View Aggregated Risk for a Portfolio
port_risk[risk.IRDeltaParallel].aggregate()
Output:
date 2020-02-13 -590.072113 dtype: float64
# Calculate Portfolio Risks within HistoricalPricingContext
from gs_quant.markets import HistoricalPricingContext
start_date = dt.date(2019, 6, 3)
end_date = dt.date(2019, 11, 18)
with HistoricalPricingContext(start_date, end_date):
port_ird_res = portfolio.calc(risk.IRDeltaParallelLocalCcy)
port_ird_agg = port_ird_res[risk.IRDeltaParallelLocalCcy].aggregate()
swap1_ird = port_ird_res[risk.IRDeltaParallelLocalCcy]['EUR3m5y']
swap2_ird = port_ird_res[risk.IRDeltaParallelLocalCcy]['EUR6m5y']
import matplotlib.pyplot as plt
plt.figure(figsize=(7, 5))
plt.xlabel('Date')
ax1 = port_ird_agg.plot(label='Portfolio')
swap1_ird.plot(label='EUR3m5y')
swap2_ird.plot(label='EYR6m5y')
h1, l1 = ax1.get_legend_handles_labels()
plt.title('IRDeltaParallel Timeseries')
plt.legend(h1, l1, loc=2)
plt.show()
Output:
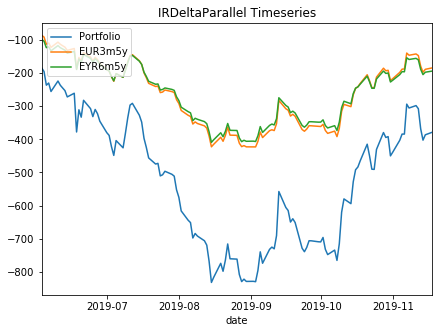
Related Content
Previous - Pricing Context
arrow_forwardNext - Scenarios
arrow_forwardWas this page useful?
Give feedback to help us improve developer.gs.com and serve you better.